I came across a firebug error, "SyntaxError: syntax error " which took me a half an hour to crack, so hopefully I can help you not waste half an hour yourself!
The bug looks like this:
I googled and found out that it might be caused by a script-tag not having a src-attribute. But all my scripts have that! So... I ran it through fiddler, and lo and behold, it turns out the page couldn't even load my script - because I was doing an old-fashioned asp.net solution and I hadn't included the scripts-directory in my locations, doh!!:
Once I did so, case was closed. So the bug was between the keyboard and monitor...
HTH others.
torsdag den 29. november 2012
tirsdag den 20. november 2012
WCF self-hosted Windows Workflow flowchart debugging
I'm looking into Microsoft Windows Workflow solutions - a really powerful tool, once you get the hang of it (it's a steep learning curve).
Testing a workflow is the easiest if you simply host the workflow within Visual Studio's own development web server. But I've found a debugging issue if you use a flowchart workflow as opposed to a sequential workflow.
In my case I was trying to step into a custom activity (a codeActivity), but no matter how I tried it, my breakpoints would not be hit. If I ran a sequential workflow, no problem, the breakpoints got hit.
The 'solution' was to simply start two instances of Visual Studio, load the solution into both and for the first one set the workflow project as the start-up project (and fire it up), and for the second one set the other project that's hitting the service (a console-application, in my case) and fire that one up. Having its own seperate managed instance ensured the workflow projects breakpoints were hit.
Another solution would be to add a 'Debugger.Break();'-statement, which would then, when hit, offer you a choice of debugging the solution. But it's far easier to just seperate the projects with two Visual Studio sessions.
HTH others.
Etiketter:
c#,
Visual Communication Foundation,
Visual Studio,
wcf,
WF,
Workflow
fredag den 21. september 2012
jquery calendar appointment time picker
I was in need of a time picker with a corresponding duration-selector; and as I couldn't find anything which suited the requirements, I decided to make it myself. So here it is for you to grab if you like. This is what i looks like:
The requirement was to select a specific time on a time ruler, so I'm drawing a ruler on a html5 canvas element and, beneath it, a ruler to select the duration. With both rulers I overlay a 'ruler-marker' div, of course absolutely positioned, and those are decorated with the jquery UI 'draggable' attribute - limited in as much as you can only move the marker within the ruler. A 'onDrag'-handler makes sure the size of the ruler-marker of the time-ruler expands or contracts with the change in duration.
It's important to incorporate the touch-punch - http://touchpunch.furf.com/ - js support for the jquery ui, if you expect the draggable-functionality to work on IOS devices.
So that's a day's work for me, hopefully it'll save you one. Here's the source:
https://docs.google.com/open?id=0B8b-I3PiuN9lMlg2UnoxQnQ5MkU
Oh, there's a 'Helperfunctions.js' involved which I don't link to, but it incorporates a 'GetAbsolutePosition()'-function that I nicked from here: http://blogs.korzh.com/progtips/2008/05/28/absolute-coordinates-of-dom-element-within-document.html
onsdag den 19. september 2012
c# and Skype integration
UPDATE 7/9/13: I heard a rumor that Skype would no longer support the below method of integration. It's probably worth your while to check it out, and thus not waste your time if that's indeed the case.
I was asked to build a windows desktop application which offered video-assistances to students who visited with our physical helpdesk, only to find no-one there to help them - wherefore they could instead perform a video-call to the first available helpdesk-staffer.
My choice in building the application was to utilize the Skype API, in as much as the audio and video part is proven and reliable, and, well, there's no sense in re-inventing the wheel. They all use Skype to make intranet calls anyway, so they're used to it and very familiar with it. And so are the students, for that matter. So that's what I did and it worked out terrific. And here's how I did it:
1) You'll have to obtain a Skype licence. So go to http://developer.skype.com/ and join the developer program of your choice, either embedded or desktop. It's not a very costly program to join. This will get you your developer key. However, you'll download a *.pem-file, but in order to program against the Skype API on windows you'll need to convert this to a *.pfx file. No biggie: use this page - https://www.sslshopper.com/ssl-converter.html - to do an online conversion, which will result in a pfx-file you can import into your c# project, as did I. P.S.: the online conversion page will have you select both a 'Private Key File:' and a 'Certificate File to Convert:'. In both fields provide your downloaded *.pem file.
2) So you've got your *.pfx licence-file now, and the next thing you'll need is the SkypeKit_VS2010.dll so you can program against the API. Specifically you'll want to download the SkypeKit version 4.3.1 - here it is: http://developer.skype.com/skypekit/releases/skypekit-4-3-hf1/release_files/skypekit/downloads/101904 (desktop version). The gz-file you'll download via the above link contains a Visual Studio 2008 and 2010 project alike, and when you build that you get the dll as a result. Alternatively you can download my source code, the Visual Studio 2010 version of the dll is in there. I do recommend download the above kit, though, there's some great example code for your perusal.
2.5) Download the Skype runtime exe-file. The api-calls you make will be executed against this runtime exe-file. The exe is in the SkypeKit you downloaded - or you can browse the 'dll'-folder in my source code and the windows x86 exe is in there. 3) You've got your licence key and your dll. Reference the dll in your project and you'll be ready to program against the API. You'll do the following:
a) reference your licence key, like this: X509Certificate2 cert = new X509Certificate2(@"C:\userprofiles\mno\My Documents\Visual Studio 2010\Projects\SkypeTest\SkypeTest\SkypeStuff\Version.pfx", @"wallen11");
b) initiate a new Skype API connection: skype = new SktSkype(this, cert, true, false, 8963);
c) declare the 'onConnect' event handler associated with the skype API connection you've just initialized. This connect-handler will handle login via your account. skype.events.OnConnect += OnConnect;
d) Launch the Skype runtime. Again, this runtime receives the commands you send to the Skype API and executes them. skype.LaunchRuntime(@"C:\yourPathToTheExe\windows-x86-skypekit.exe", true);
e) ... And connect: skype.Connect();
f) The connect-command will trigger the onConnect-handler, which takes care of our logging into Skype:
public void OnConnect(object sender, SktEvents.OnConnectArgs e)
{
if (e.success)
{
account = skype.GetAccount(
account.LoginWithPassword(
}
else
{
throw new Exception("IPC handshake failed with: " + e.handshakeResult + "\r\n");
}
}
4) Let's assume you've got connected to Skype, i.e. your desktop application is connected to the Skype network. Now it's a matter of making a call from the desktop application. This is where it gets a bit tricky. Your only - for now - option in calling other Skype contacts is to emulate a previous conversation you've had with them. You can do something akin 'SkypeCall call = new SkypeCall("SkypeAccountIWantToCall"); call.Ring();'.
What you must do is first get a list of your account's previous conversations:
conversationList = skype.GetConversationList(SktConversation.LIST_TYPE.ALL_CONVERSATIONS);
... and from one of those conversations you'll retrieve the list of participants:
SktParticipant.List parts = selectedConversation.GetParticipants(SktConversation.PARTICIPANTFILTER.OTHER_CONSUMERS);
List
foreach (SktParticipant part in parts)
{
names.Add(part.P_IDENTITY);
}
// call the contact
conv.RingOthers(names, true, "");
There's a bit more to it than that - such as handling video, and checking for contact availability. I'll refer to my sample code. What I do is this, I get a list of the previous conversation and sort through these. I add the conversations by distinct users to an invisible listbox. A 'call' button traverses the conversations and checks availability of the participants; first available helpdesk employee gets the honors. That's perhaps not the most elegant fashion, the invisible listbox-thing, in my defence I was in a hurry and leaned heavily on the tutorial code provided by Skype.
As always here's a link to the source code of my effort:
https://docs.google.com/open?id=0B8b-I3PiuN9lMXNkTllyU1dGVms
Not documented terribly well, but, as always, get back to me if there's anything I can explain better, or if you need help in general.
Hope it helps! Best of luck in your coding efforts.
onsdag den 29. august 2012
Slow onClick on iPhone and iPad
I was building a javascript-powered zip-code registration web page, with a numeric display which would be used to enter the zip-codes of guests at a company grand social function, to later determine where they came from. The screenshot below is what I came up with:
Your basic numeric display with digits, a 'clear' button and a 'undo latest' button. Upon entering a 4-digit zip, the 'display' automatically clears, ready for a new one.
But the app was to run exclusively as a web-app within an iPad, and what I found was that the onClick handlers I'd attributed to the buttons, which are actually DIVs, were slow to trigger, on both my version 1 and version 2 iPad. A bit of googling suggested I went with the touchstart event instead; which worked beautifully, the javascript associated with the events executed quickly and beautifully.
So to recap, in the $(document).ready(function () -section I did this:
$('.digit').bind("touchstart", function () {
var clickedValue = this.getAttribute("value");
// do something with the button's digit value here
}
... where '.digit' corresponds with the class I give my DIVs:
9
Give me a shout if you want the code, it's a Visual Studio 2010 c# web application. HTH someone in a similar bind.
Etiketter:
ios,
ipad,
iphone,
jquery,
onClick,
onclick slow,
touchStart
onsdag den 15. august 2012
Prevent zooming on iPhone and iPad web apps
Had to google more than I thought I'd have to in regards to this one, so here's a blog entry to hopefully help others out.
To disallow the user to zoom in and out on a web page as displayed on an iPad or iPhone, add this tag to the meta-tags section of the page header:
This will prevent the user from zooming in or out on the screen. I saw a range of other attempts to prevent this, using javascript and such, but the above is your best option.
tirsdag den 10. juli 2012
Editing PDF form on the web
I was asked to make a PDF form editable on the web. The HR department already had the form, now they wanted me to make it editable via an url.
The solution involves
- setting up a database to hold the PDF form data,
- making the PDF form submittable to a web page,
- utilizing the iTextSharp-library to parse the submitted PDF form
Setting up the database involves creating a table with columns for every field in the PDF-form you need stored - likely all of them. Also there must be a primary key in the form of an auto-incremented value or a global unique identifier. Having set up the database, allow the PDF form to be submittable to a web page. In Adobe Acrobat Pro, go to the "Forms -> Add or Edit Fields" and add a PDF button with the text "save" on it. Define a button action for the mouse-up event; specifically, a "submit form" action (*). The properties for the action should look akin to this:
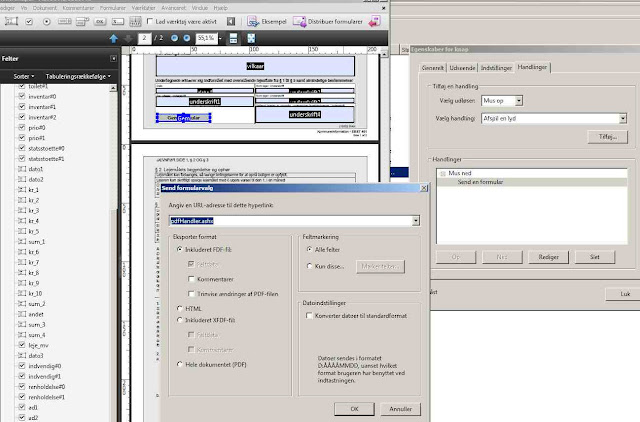
*) I'm using a Danish version of Acrobat Pro, so the menus and texts may slightly different from the above.
I.e. the form submits into a generic handler "pdfHandler.ashx" when it is clicked (the mouse-down action). The handler's default "ProcessRequest" function looks like this:
---
Basically what takes place here, is that an iTextSharp pdf-reader is instantiated from a HTTPRequest which is the submitted PDF document. Using LINQ to SQL, a database object corresponding to the PDF form (recall we just created the database and its table with columns for all the PDF form fields) is populated from the PDF form (the 'GetFieldValue' function of the iTextSharp library) and stored to the database via LINQ to SQL.
The "New" option is relatively straight forward, in as much as the LINQ to SQL functionality itself works out to insert a new auto incremented integer primary key, if you're - as am I in this case - going with auto-integers for database keys. The "Edit" option is more of a challenge - here we'll have to inject the primary key into the PDF form, before allowing the generic handler to take over and grap this value when the form is submitted. The way to do this is so:
In the edit-button click function, do this:
---
What happens here is that we instantiate an iTextSharp PDF-reader and use the "GETPDFField"-method to achieve a reference to all the form fields in this PDF. THe "SetFormData" function simply fills in these form fields (like so; "af.SetField("ident", lejer.id.ToString());") - including a hidden "ident" field on the form. Once the form fields have been populated, we'll redirect into the form by changing the page headers and response contenttype, as written.
Hope the above helps! All the references to 'fields', 'forms' and such can get confusing, so be sure to get in touch with me if anything needs explaining further.
The solution involves
- setting up a database to hold the PDF form data,
- making the PDF form submittable to a web page,
- utilizing the iTextSharp-library to parse the submitted PDF form
Setting up the database involves creating a table with columns for every field in the PDF-form you need stored - likely all of them. Also there must be a primary key in the form of an auto-incremented value or a global unique identifier. Having set up the database, allow the PDF form to be submittable to a web page. In Adobe Acrobat Pro, go to the "Forms -> Add or Edit Fields" and add a PDF button with the text "save" on it. Define a button action for the mouse-up event; specifically, a "submit form" action (*). The properties for the action should look akin to this:
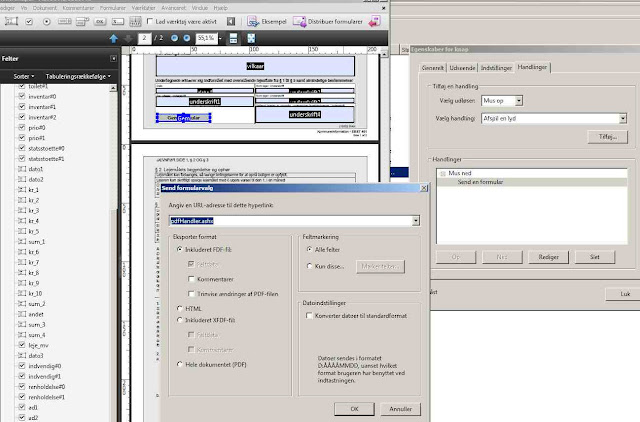
*) I'm using a Danish version of Acrobat Pro, so the menus and texts may slightly different from the above.
I.e. the form submits into a generic handler "pdfHandler.ashx" when it is clicked (the mouse-down action). The handler's default "ProcessRequest" function looks like this:
---
public void ProcessRequest(HttpContext context)
{
HttpRequest pdfRequest = HttpContext.Current.Request;
System.IO.Stream istream = HttpContext.Current.Request.InputStream;
FdfReader fdf = new FdfReader(istream);
// eksisterende?
if (!string.IsNullOrEmpty(fdf.GetFieldValue("ident")))
{
DataClassesDataContext dbContext = new DataClassesDataContext();
int id = Convert.ToInt32(fdf.GetFieldValue("ident"));
lejere lejer = dbContext.lejeres.Single(foo => foo.id.Equals(id));
lejer.ad1 = fdf.GetFieldValue("ad1") == null ? string.Empty : fdf.GetFieldValue("ad1").ToString();
// populate all database object properties
dbContext.SubmitChanges();
}
else
{
#region new lejer
try
{
DataClassesDataContext dbContext = new DataClassesDataContext();
lejere lejer = new lejere()
{
ad1 = fdf.GetFieldValue("ad1") == null ? string.Empty : fdf.GetFieldValue("ad1").ToString(),
ad2 = fdf.GetFieldValue("ad2") == null ? string.Empty : fdf.GetFieldValue("ad2").ToString(),
// populate all database object properties
};
if (string.IsNullOrEmpty(fdf.GetFieldValue("lejernavn1")) && string.IsNullOrEmpty(fdf.GetFieldValue("lejernavn2")))
{
lejer.lejernavn1 = "Uudfyldt";
lejer.lejernavn2 = "Uudfyldt";
}
dbContext.lejeres.InsertOnSubmit(lejer);
dbContext.SubmitChanges();
}
catch (Exception ex)
{
throw ex;
}
#endregion
}
HttpContext.Current.Response.Redirect("Default.aspx", true);
}
---{
HttpRequest pdfRequest = HttpContext.Current.Request;
System.IO.Stream istream = HttpContext.Current.Request.InputStream;
FdfReader fdf = new FdfReader(istream);
// eksisterende?
if (!string.IsNullOrEmpty(fdf.GetFieldValue("ident")))
{
DataClassesDataContext dbContext = new DataClassesDataContext();
int id = Convert.ToInt32(fdf.GetFieldValue("ident"));
lejere lejer = dbContext.lejeres.Single(foo => foo.id.Equals(id));
lejer.ad1 = fdf.GetFieldValue("ad1") == null ? string.Empty : fdf.GetFieldValue("ad1").ToString();
// populate all database object properties
dbContext.SubmitChanges();
}
else
{
#region new lejer
try
{
DataClassesDataContext dbContext = new DataClassesDataContext();
lejere lejer = new lejere()
{
ad1 = fdf.GetFieldValue("ad1") == null ? string.Empty : fdf.GetFieldValue("ad1").ToString(),
ad2 = fdf.GetFieldValue("ad2") == null ? string.Empty : fdf.GetFieldValue("ad2").ToString(),
// populate all database object properties
};
if (string.IsNullOrEmpty(fdf.GetFieldValue("lejernavn1")) && string.IsNullOrEmpty(fdf.GetFieldValue("lejernavn2")))
{
lejer.lejernavn1 = "Uudfyldt";
lejer.lejernavn2 = "Uudfyldt";
}
dbContext.lejeres.InsertOnSubmit(lejer);
dbContext.SubmitChanges();
}
catch (Exception ex)
{
throw ex;
}
#endregion
}
HttpContext.Current.Response.Redirect("Default.aspx", true);
}
Basically what takes place here, is that an iTextSharp pdf-reader is instantiated from a HTTPRequest which is the submitted PDF document. Using LINQ to SQL, a database object corresponding to the PDF form (recall we just created the database and its table with columns for all the PDF form fields) is populated from the PDF form (the 'GetFieldValue' function of the iTextSharp library) and stored to the database via LINQ to SQL.
The "New" option is relatively straight forward, in as much as the LINQ to SQL functionality itself works out to insert a new auto incremented integer primary key, if you're - as am I in this case - going with auto-integers for database keys. The "Edit" option is more of a challenge - here we'll have to inject the primary key into the PDF form, before allowing the generic handler to take over and grap this value when the form is submitted. The way to do this is so:
In the edit-button click function, do this:
---
int lejerId = Convert.ToInt32(LejereDropDown.SelectedValue);
DAL.lejere lejer = dbContext.lejeres.Single(foo => foo.id.Equals(lejerId));
PdfReader reader = null;
AcroFields af = null;
PdfStamper ps = GetPDFFields(ref reader, ref af);
SetFormData(ref af, lejer);
// forget to close() PdfStamper, you end up with
// a corrupted file!
ps.Close();
if (reader != null)
{
Response.AddHeader("Content-Disposition", "inline;filename=lejekontrakt.pdf");
Response.ContentType = "application/pdf";
}
DAL.lejere lejer = dbContext.lejeres.Single(foo => foo.id.Equals(lejerId));
PdfReader reader = null;
AcroFields af = null;
PdfStamper ps = GetPDFFields(ref reader, ref af);
SetFormData(ref af, lejer);
// forget to close() PdfStamper, you end up with
// a corrupted file!
ps.Close();
if (reader != null)
{
Response.AddHeader("Content-Disposition", "inline;filename=lejekontrakt.pdf");
Response.ContentType = "application/pdf";
}
What happens here is that we instantiate an iTextSharp PDF-reader and use the "GETPDFField"-method to achieve a reference to all the form fields in this PDF. THe "SetFormData" function simply fills in these form fields (like so; "af.SetField("ident", lejer.id.ToString());") - including a hidden "ident" field on the form. Once the form fields have been populated, we'll redirect into the form by changing the page headers and response contenttype, as written.
Hope the above helps! All the references to 'fields', 'forms' and such can get confusing, so be sure to get in touch with me if anything needs explaining further.
Etiketter:
Adobe Acrobat Pro,
asp.net,
c#,
editable,
pdf
tirsdag den 3. juli 2012
Unsafe code in App_Code folder
I ran into an issue where my Visual Studio 2010 Professional reported "CS0227: Unsafe code may only appear if compiling with /unsafe".
But I had already checked the appropriate checkbox in the solution properties dialog!
Turns out the problem was having the unsafe code reside in the App_Code folder. Moving it to a different location solved the problem.
But I had already checked the appropriate checkbox in the solution properties dialog!
Turns out the problem was having the unsafe code reside in the App_Code folder. Moving it to a different location solved the problem.
mandag den 4. juni 2012
Live c# video streaming webcam server and client
I wanted to capture live video from a webcam and stream it directly to a client application. This was thought to be for a robot which would stream video from its webcam eyes and allow me to see what the robot sees, but really it would be possible to build a cheap DIY home surveillance network around this.
The VS 2010 c# solution holds two projects, one commandline project for the server and one windows forms project for the client.
The server stream not video, but rather a series of captures images, but in presenting these images in a rapid fashion the end result will seem like a naturally flowing video stream. The images made available to a connecting client IP by an initialized WCF service, namely via a tcp/ip binding. The images capture is done by utilizing the AForge framework to access an available webcam. The images are saved to disk and not to memory, as I wanted to retain the option of going through them again at a later point. The last captured image is always kept in memory, to be delivered to the calling client as the WCF's 'getWebCamImage()' method is invoked.
I set the web cam framerate to 15 frames per second, which is fine enough to moving images. The maximum number of images the human eye can distinguish is 30 per second, so I'm told, so 15 images per second makes for a comfortably video experience.
The client creates an instance of the WCF service. A timer is set to call the service and return an image-stream, which is placed into a standard winforms picturebox. In the example code the timer is set at 100 milliseconds, which will yield only 10 images per second - thus not the 15 frames which are actually captured, I did this to perserve bandwidth but in reality it should be perfectly possible to call the timer 15 times per second. The viewing experience is nice enough just the same.
Check out the source code below if you're in need of something like this. Please bear with all the references to 'robot this' and 'robot that', the functionality was meant for a robot control project. The WCF service runs localhost, but you would of course in due time seperate the client from the server and allow the service to run via an external IP, firewall-configured to the port of your choice and not my standard port no 8733.
Link to solution source code
Let me know if there's anything I can do to help you in implementing the project, if it gives you any trouble.
Hope this helps you!
Buy me a coffee
The VS 2010 c# solution holds two projects, one commandline project for the server and one windows forms project for the client.
The server stream not video, but rather a series of captures images, but in presenting these images in a rapid fashion the end result will seem like a naturally flowing video stream. The images made available to a connecting client IP by an initialized WCF service, namely via a tcp/ip binding. The images capture is done by utilizing the AForge framework to access an available webcam. The images are saved to disk and not to memory, as I wanted to retain the option of going through them again at a later point. The last captured image is always kept in memory, to be delivered to the calling client as the WCF's 'getWebCamImage()' method is invoked.
I set the web cam framerate to 15 frames per second, which is fine enough to moving images. The maximum number of images the human eye can distinguish is 30 per second, so I'm told, so 15 images per second makes for a comfortably video experience.
The client creates an instance of the WCF service. A timer is set to call the service and return an image-stream, which is placed into a standard winforms picturebox. In the example code the timer is set at 100 milliseconds, which will yield only 10 images per second - thus not the 15 frames which are actually captured, I did this to perserve bandwidth but in reality it should be perfectly possible to call the timer 15 times per second. The viewing experience is nice enough just the same.
Check out the source code below if you're in need of something like this. Please bear with all the references to 'robot this' and 'robot that', the functionality was meant for a robot control project. The WCF service runs localhost, but you would of course in due time seperate the client from the server and allow the service to run via an external IP, firewall-configured to the port of your choice and not my standard port no 8733.
Link to solution source code
Let me know if there's anything I can do to help you in implementing the project, if it gives you any trouble.
Hope this helps you!
torsdag den 10. maj 2012
Recommending a free subversion service
If you're in need of a free SVN service to hold your code, I can recommend trying Assembla. I've been with them for a few months and so far it's been very smooth sailing. Their free plan for non-commercial code hosting and versioning is great for my needs. Couple with the - also free - Ankh Visual Studio SVN plugin, and you'll never loose code or sleep again.
And I'm not affiliated with them in any way, suffice to say.
And I'm not affiliated with them in any way, suffice to say.
tirsdag den 1. maj 2012
could not create type webservice
A little reminder, if you encounter the "could not create type 'whatever'" when trying to access an asmx web service you created, this could be caused by the virtual directory not being marked as an IIS web application - like this:
In IIS 7, right-click the virtual directory and select "Convert to application".
In IIS 7, right-click the virtual directory and select "Convert to application".
fredag den 30. marts 2012
Microsoft Speech with non-english languages
I had a horrid time trying to get the Microsoft text-to-speech to work with my native Danish language. So here's how I made it work. Hope it'll help you save a few hours.
The target is a C# .net 3.5 console application. Later I'll work out how to use it from a web-server.
Key is using the same version numbers for all components. Download and install the following, in the same order as below:
- Microsoft Speech platform runtime 11.0 - http://www.microsoft.com/download/en/details.aspx?id=27225 (select the x86 og x64 platform according to your OS)
- Microsoft Speech platform SDK 11.0 - http://www.microsoft.com/download/en/details.aspx?id=27226 (again, select the x86 og x64 platform according to your OS)
Having downloaded and installed the above, now's the time to select your language of choice from the language download section: http://www.microsoft.com/download/en/details.aspx?id=27224
Download one or more of the language files with the "TTS" in the filenames (short for 'text-to-speech'). The other runtime languages are for speech recognition, not text-to-speech.
Now, in your Visual Studio, create a new console application.
Important steps:
- Set the deployment target CPU architecture to the same version of the microsoft components you downloaded. So if your OS is x86, and you thus downloaded the x86 microsoft speech components, set the deployment target as 'x86'.
- Reference the Microsoft.speech DLL from the C:\Program Files\Microsoft SDKs\Speech\v11.0\Assembly-folder.
All set, then. Here's a sample Main()-method:
The target is a C# .net 3.5 console application. Later I'll work out how to use it from a web-server.
Key is using the same version numbers for all components. Download and install the following, in the same order as below:
- Microsoft Speech platform runtime 11.0 - http://www.microsoft.com/download/en/details.aspx?id=27225 (select the x86 og x64 platform according to your OS)
- Microsoft Speech platform SDK 11.0 - http://www.microsoft.com/download/en/details.aspx?id=27226 (again, select the x86 og x64 platform according to your OS)
Having downloaded and installed the above, now's the time to select your language of choice from the language download section: http://www.microsoft.com/download/en/details.aspx?id=27224
Download one or more of the language files with the "TTS" in the filenames (short for 'text-to-speech'). The other runtime languages are for speech recognition, not text-to-speech.
Now, in your Visual Studio, create a new console application.
Important steps:
- Set the deployment target CPU architecture to the same version of the microsoft components you downloaded. So if your OS is x86, and you thus downloaded the x86 microsoft speech components, set the deployment target as 'x86'.
- Reference the Microsoft.speech DLL from the C:\Program Files\Microsoft SDKs\Speech\v11.0\Assembly-folder.
All set, then. Here's a sample Main()-method:
static void Main(string[] args)
{
// Initialize a new instance of the SpeechSynthesizer.
SpeechSynthesizer synth = new SpeechSynthesizer();
// select your installed voice
synth.SelectVoiceByHints(VoiceGender.NotSet, VoiceAge.NotSet, 0, new CultureInfo("da-dk"));
// important to set the output to the default device, or no sound will be heard.
synth.SetOutputToDefaultAudioDevice();
// speak a phrase
synth.Speak("patter der batter");
}
tirsdag den 27. marts 2012
A powershell printing application
Here's a powershell application (with a winforms GUI) which allows a user to select a number of printers retrieved from an Active Directory server (via ldap) and install them.
Link to zip-file
The code is a mess, there're a lot of references no longer needed and unused functions which should be removed, but maybe it'll be helpful to some.
I used the free PrimalForms 2011 40-days preview to code and debug the application, I recommend it highly.
Link to zip-file
The code is a mess, there're a lot of references no longer needed and unused functions which should be removed, but maybe it'll be helpful to some.
I used the free PrimalForms 2011 40-days preview to code and debug the application, I recommend it highly.
mandag den 26. marts 2012
A JQuery Mobile mini-survey
Here's a Jquery Mobile mini survey implementation.
It's a C# asp.net web-application. Uses Linq-to-Xsd. The survey is defined in an xml-file, with a simple implementation of branching based on the selected response. The survey-pages - one question per page - are formed dynamically from the definition file. 3 different type of questions are offered; Yes/no on/off-style questions, radio-group questions (single selection), and sliders.
No security is implemented, there's only a cookie write/read to ensure the user doesn't answer the survey twice.
Link to zip-file
Hope it's a starting point for some.
It's a C# asp.net web-application. Uses Linq-to-Xsd. The survey is defined in an xml-file, with a simple implementation of branching based on the selected response. The survey-pages - one question per page - are formed dynamically from the definition file. 3 different type of questions are offered; Yes/no on/off-style questions, radio-group questions (single selection), and sliders.
No security is implemented, there's only a cookie write/read to ensure the user doesn't answer the survey twice.
Link to zip-file
Hope it's a starting point for some.
fredag den 23. marts 2012
Bind input type range change event
Took me forever to find out how to bind the input type="range" element's change-handler in JQuery. This is what I came up with, which works for me with JQuery 1.6.4:
$(document).ready(function () {
});
Hope this helps somebody.
$(document).ready(function () {
$('[type=range]').each(function () {
$("#" + $(this).attr("id")).bind("change", function (event, ui) {
alert(event.target.value);
});
});
$("#" + $(this).attr("id")).bind("change", function (event, ui) {
alert(event.target.value);
});
});
Hope this helps somebody.
Abonner på:
Opslag (Atom)